In previous blog you learned how to access the VBA Editor and write a simple script. In this Blog you will learn a few more basic scripts, including the one that allow you to get input from the user. You will also learn a little bit about variables, which are used to store information, so you can use it when you give feedback. You will get a preview of how to use some of the same scripts to get other kinds of input, such as answers to short-answer questions. Finally, you will learn some details about running your scripts and assigning them with buttons, including how to assign a button with more than one script.
- Variables and Getting Input
Earlier, we have used MsgBox to pop up a message on the screen. You can use a similar box to get input from your users. The only difference is that the new dialogue box will have a option for your users to type something. We’ll start with something simple: asking for the User’s name.
Sub YourName()
userName = InputBox(Prompt:="Type your name", _
Title:="Input Name")
End Sub
There are a few important things about this simple procedure. First, pay attention to the space and underscore at the end of the second line. The last three characters on the second line are comma, space, and underscore.

Without the space, the computer won’t recognize the underscore that follows. The underscore is a special VBA character that tells VBA that what is on the next line is part of this line. Therefore, that entire line could have been written on one line without the underscore, just like below:
userName = InputBox(Prompt:="Type your name", Title:="Input Name")
The underscore simply allows you to divide long lines so you don’t have to scroll to the right to see what is on each line. The next thing that is important about this small piece of code is that it uses a variable: userName. Since we don’t do anything with the variable at this point, it is not terribly interesting, but we should note a few things about variables. Variables are places to store information. You can think of them as STORAGE boxes in the computer’s memory and these variables change values. That is, you can take something out of a box and put something else into the box.
Next important thing is the equal sign (=) and it is not a statement of equality. It is an assignment operator. It says, take the value on the right side and store it in the variable named on the left side. Therefore, the previous procedure is not a statement of algebraic fact; it is an action.
In the YourName procedure, we have used the variable userName. What we tried to do is: Take whatever the user types in the InputBox and put it into a variable called userName. Later, we will want to use the name so we will get it out of the userName box when we are ready.
- Variable Declarations
For a variable to be useful, you often need to declare it. Although it is not necessary to declare all variables, it is good practice to do so. Declaring a variable does two things for you: It tells the computer which procedures are allowed to know about the variable (scope), and it tells the computer what kind of information (type) the variable can hold. Declaring a variable is very easy. You do it with the Dim statement:
Dim userName
This line tells the computer that you want a storage box called userName to store some information. The most important part about the Dim statement is where to put it. You have two choices: You can put it at the beginning of your procedure (right after the Sub statement) or at the beginning of your module (before any Sub statements). A Dim statement at the beginning of a module means that every procedure in the module can access that variable. That is, the scope of the variable is the entire module.
Alternatively, if you put the Dim statement at the beginning of the procedure (right after the Sub statement), only that procedure can use the variable; that is, the scope of the variable is the procedure.
Remember: A module can have more than one procedure.
So to sum it up, its totally based on scripter’s requirement where he decides to place the Dim statements and so will be the scope of the variable. For this procedure, let’s put Dim statement on top of all procedures in a module and at later point in the blog we will write another procedure to access the value stored in this variable.
Dim userName
Sub YourName()
userName = InputBox(Prompt:="Type your name", _
Title:="Input Name")
End Sub
- Variable Types
Variables are of certain types. That is, certain variables can hold certain kinds of information. If you don’t tell the computer what kind of information the variable is holding in advance, it will figure it out. In the YourName procedure, the function InputBox always returns a variable of type String (a String is text), so VBA will figure out that userName is a String. However, it is a good idea to tell the computer that you want userName to be of type String. You can do this by changing the earlier Dim statement to:
Dim userName As String
This Dim statement not only tells the computer that we want a variable called userName, but it also tells it what kind of information that variable can hold. In this case, our variable will hold a String (i.e., text) of up to 65,536 characters long.
Note: When you type a space after As , VBA editor will use Intellisense to try to suggest things for you to type with a little box that pops up.
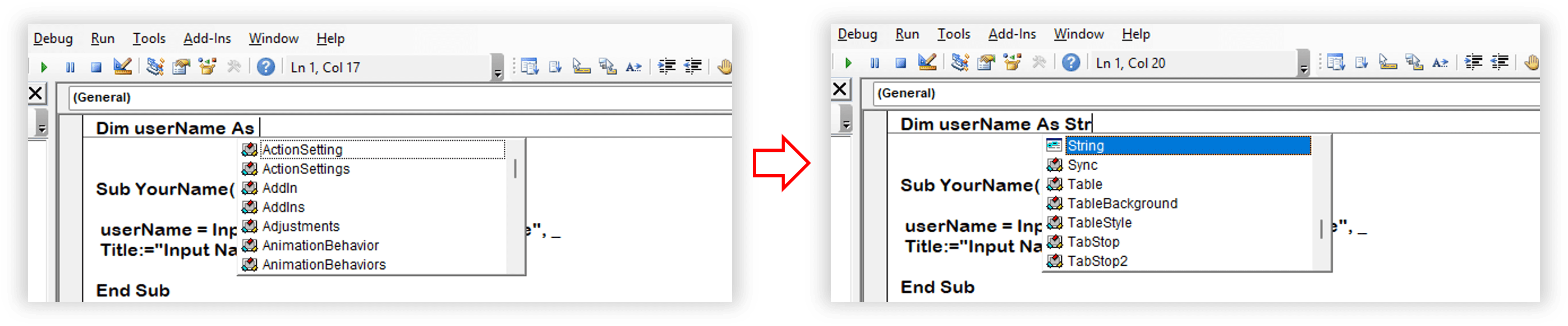
This box contains all the things that you can type now. Boxes like this will pop up frequently. If you know what you want to type, just ignore the box. If you’re not sure what you want to type, scroll through the list to see the possibilities. Actually there are about 300 of them, but there are just a few that you will care about now. Common types you will use are:
Common Variable Types
Boolean | True or False values |
Integer | Any integer from –32,768 to 32,767 |
Long | Any integer from –2,147,483,648 to 2,147,483,647 |
Shape | Any PowerPoint shape (such as those things that can be drawn with the Draw tools) |
Single | Non-integers (i.e., numbers with something after the decimal point, such as 3.14 and 98.6) |
String | Any text up to 65,536 characters long |
Object | Any object |
Now, we are ready to put it all together with a Dim statement and two procedures:
Dim userName As String
Sub YourName()
userName = InputBox(prompt:="Type your name", _
Title:="Input Name")
End Sub
Sub greetUser()
MsgBox "Good Morning, " & userName
End Sub
The first procedure could be associated with a button on the first slide, and the second procedure could be associated with a button on a later slide. The result would be that when the first button was pressed, the student would be asked to “ Type your name.” If the student types “Krishna,” when the second button is pressed, a message would pop up on the screen saying, “You are doing well, Krishna.”
The & (ampersand) character used in the MsgBox procedure is for concatenation of strings; i.e., the two strings “You are doing well,” and whatever is stored in the variable userName (in this case “Krishna”) are joined together to make one string, “You are doing well, Krishana,” which is displayed in the box on the screen. Watch carefully for punctuation and spacing. If, for example, you don’t put the space after the comma, then the userName will be right next to the comma without a space, as in: “You are doing well,Krishna.”
If you have forgotten how to assign your buttons to a procedure, look back in this blog post.
In later Blog Posts, you’ll learn how to check what was typed to make sure it is OK, as well as how to force the user to click on the button.
- Calling a Procedure from Another Procedure
Not all procedures are tied directly to buttons. Many procedures are designed to do part of what you want a button to do. These procedures are called/triggered from other procedures. For example, let’s consider two procedures we have already written: YourName and greetUser
Dim userName As String
Sub YourName()
userName = InputBox(prompt:="Type your name", _
Title:="Input Name")
End Sub
Sub greetUser()
MsgBox "Good Morning, " & userName
End Sub
You could assign a button with each of these procedures, so the users click on the first button to type their names and click on the other button to greet themselves. What if we want to greet the user right away, right after they have typed a name? We could write another procedure that calls the two previous procedures:
Sub YourNameWithGreet()
YourName
greetUser
End Sub
No buttons have to be associated with YourName or greetUser. Create a button and assign it with YourNameWithGreet, and that is all you need. The button will activate YourNameWithGreet. When YourNameWithGreet starts to execute, it will see the first line: YourName. That signals it to run the YourName procedure. When it finishes the YourName procedure, it will run greetUser. Your module will look like below,
